Browser extension development, simplified.
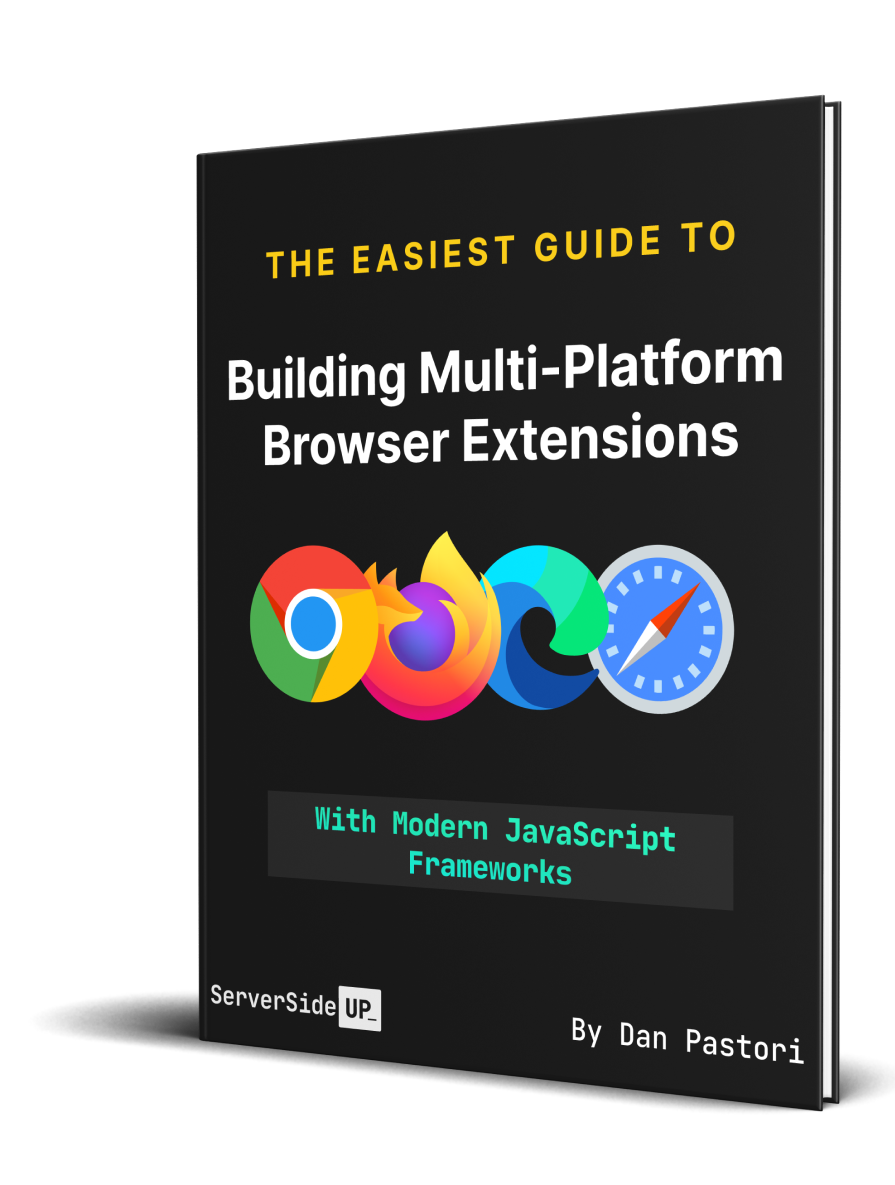
Skip the time reading outdated, unsupported documentation from multiple sources and get started with development immediately.
I wrote this book to be the guide I wish I had when we were creating Bugflow's browser extension. Even with 15 years of JavaScript experience, I struggled sifting through the browser extension documentation.
I kept running into questions like:
- How do I structure my extension?
- Where should I add this feature?
- How should do I communicate to my background script?
- What is a background service worker.
- Why doesn't this work in Firefox?
- How do I interact with the page?
All these questions will be answered, along with a lot more tutorials about what you can add to your browser extension.
Whether you are just curious on how browser extensions work or have an extension you want to create, we will guide you through the process every step of the way.
I've also created vanilla Javascript, Vue 3, and React based templates to help. Upon purchase of the book, you can immediately pull one or all of these templates from GitHub and get started.
As long as you have a familiarity with JavaScript, the development will be the easy part. The book will guide you through the challenging sections. By the end, you will have an extension works on all four major browsers: Chrome, Firefox, Edge and Safari.
Since 2011, we've run a successful bootstrapped development consulting agency called 521 Dimensions. Working with a variety of clients and tech stacks, we've gained valuable, real-life expereince that we share on our blog, ServerSideUp.
We've also created a few products, including Bugflow, a bug tracking tool for developers and wrote another book about developing web and mobile apps from the same codebase. While writing the browser extension for Bugflow, we got inspired to write this book.
Giving back is a huge piece of our beliefs. We are passionate creators of open-source software and have created and contributed to a variety of projects. Check out our GitHub and join our Discord channel to get involved!
Here's a sneak peak of what we will cover in this book.
- Building a Content Script
- Content scripts are powerful tools that live on a web page, have access to the DOM, and communicate with your extension.
- Where to add features
- There are multiple areas in your browser extension to add code. Learn which one is best for what you need.
- Create a Popup View
- A popup view is the most familiar way to interact with a browser extension. We will create one using both JS and Vue 3.
- Extension Pages
- Did you know you can have a dedicated page to run your extension? We will make one. You can fit a whole app in this page if you wanted!
- Adding Context Menus
- Want to allow your user to right click and interact with your extension? We've got you covered.
- User Authentication
- Authenticating a user with your browser extension is challenging. I'll help you get this enabled.
- Local Storage
- Set state, cache data, and interact with your browser's local storage with ease.
- Using Environment Variables
- Such a simple way to add that extra developer experience when designing and building your browser extension.
- Manifest V3
- Use the latest and greatest Manifest V3 for your extensions. Supported by every browser!
Start immediately with the framework of your choice
Upon purchase, you can immediately pull down a multi-platform browser extension template and get started. I've made a template for those who prefer Vanilla JS, Vue 3, or React.
Each template is fully functional, documented, and ready to start development. You will get an extension with the following features:
- Preconfigured Popup, Content Script, Background Script, and Extension Page.
- Extension messaging configured and ready to expand
- Background tasks (alarms)
- Tab listeners
- Webpage to extension messaging
- Local storage examples
- Context Menu
- Keyboard Shortcut
All features come documented and ready to expand upon. Just pull the template and start adding your own code!
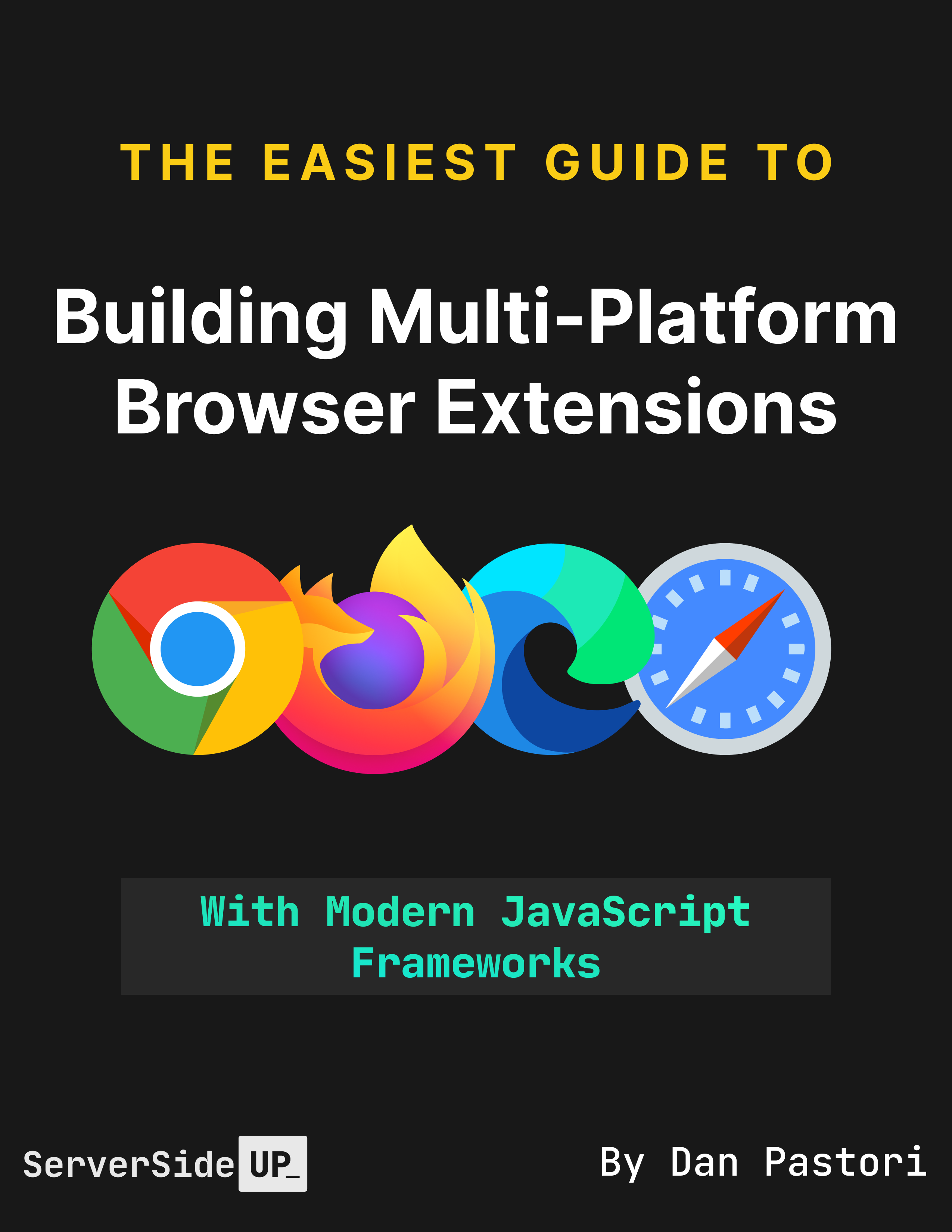
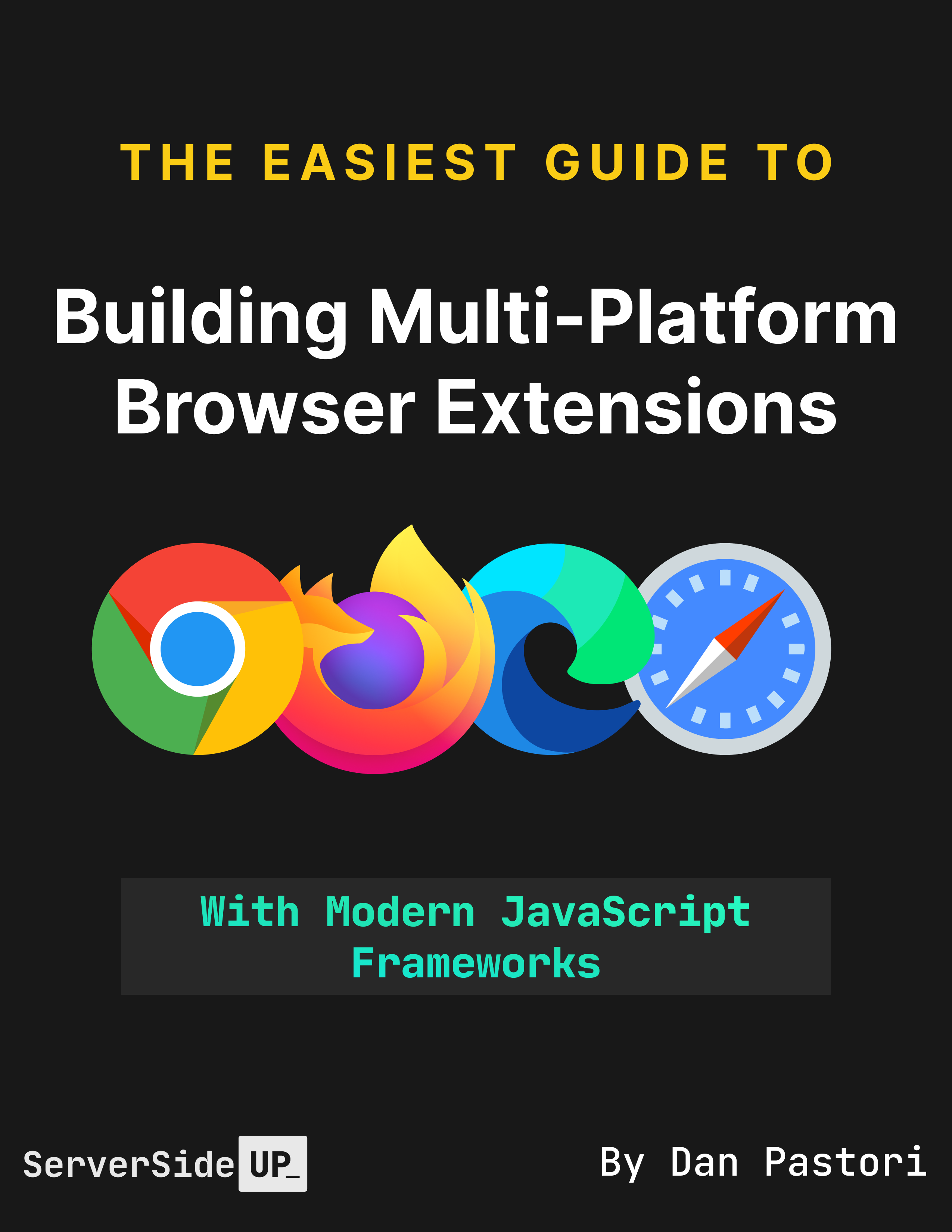
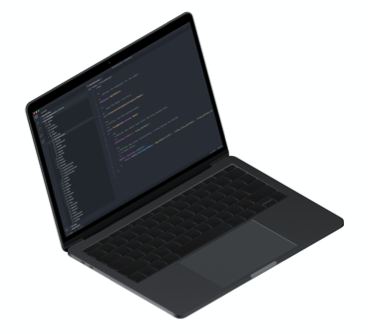
You'll get instant access after your purchase. Here’s how it works.
The book is only available in digital form (PDF).
After your purchase, Lemon Squeezy will email a receipt with where you can click "generate an invoice".
The book is available in English, but you can use Google Docs to translate the PDF into your native language.
Just shoot us an email at [email protected]